How To Remove Backgrounds From Images With Python
Just eight lines of code.
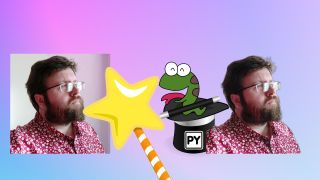
Python is a multi-purpose programming language. It does many things, from creating web apps to checking out who is on the International Space Station with a Raspberry Pi Pico W.
Python is easy to learn due to it being easy to read. What makes Python multi-purpose are modules of prewritten code, sometimes referred to as “libraries”. These modules bring in new functionality, for example RPi.GPIO enables Python to control the GPIO of the Raspberry Pi.
In this how to, we will use two Python modules to create a GUI application that will remove the background from an image. The first module, rembg from Daniel Gatis will remove the background from any image presented to it. The second module, easygui provides a means to create dialogs and menus using the operating system’s toolkit. So a file open / save dialog box will look exactly like those used in many other applications.
To take this project further, you can make your applications by packaging the project code into a single executable file.
Setting Up
Before we write a line of code we need to get everything in order. First we will create a folder to store the images that we will be working with. We will then open a Python editor for the coding part of the project.
1. Create a new folder on your desktop called rembg.
2. In the folder place an image that you wish to remove the background from.
3. Open your preferred Python editor, we prefer Thonny as it provides a simple user interface. Follow this guide to install Thonny.
Stay On the Cutting Edge: Get the Tom's Hardware Newsletter
Get Tom's Hardware's best news and in-depth reviews, straight to your inbox.
Installing the Python Modules
In order to use Rembg we first need to download and install its Python module. This can be handled via Thonny’s built-in package manager, or via Python’s packaging tool, pip.
Installing via Thonny
1. Click on Tools >> Manage Packages.
2. Search for rembg and click Search on PyPi. Select rembg from the returned list.
3. Click on Install to download and install rembg for Python. We have already installed rembg on our system, hence the uninstall button.
4. Repeat steps 2 and 3, but this time search for and install easygui.
Installing via Pip
If you are using another Python editor, you will need to install the Python packages using pip.
1. Open a Command Prompt and install rembg. Press Enter to start the process.
pip install rembg
2. Install easygui using pip. Easygui provides a basic user interface for file open and save operations.
pip install easygui
Writing the Code
The code is essentially very simple, with just eight lines of Python we can remove the background from any image. The underlying modules, rembg and easygui will be doing all of the heavy lifting for us.
1. From the rembg module import the remove class. This is what we shall use to remove the background.
from rembg import remove
2. From the Python Imaging Library (PIL), import Image. PIL is a powerful module that contains many different options for creating and working with images and image streams.
from PIL import Image
3. Import the easygui module and create a reference to it as “eg”. Easygui is our GUI for basic file operations. Renaming it to “eg” makes it easier to work with.
import easygui as eg
4. Create an object, input_path and use it to store the path and name of a file that we wish to remove the background from. Using easygui’s file open dialog box, we give the dialog a title, to explain what it is for. The chosen file and its path are stored to the input_path object.
input_path = eg.fileopenbox(title='Select image file')
5. Create an object, output_path and use easygui’s file save dialog box to capture the file path and save it to the object.
output_path = eg.filesavebox(title='Save file to..')
6. Create an object, input and use it to open and store the image via PIL’s Image.open function.
input = Image.open(input_path)
7. Use rembg to remove the background from the image.
output = remove(input)
8. Save the new image using the file path stored in output_path.
output.save(output_path)
9. Save the code as background_remover.py.
10. Run the code by clicking on the Run button.
11. Select the image that you wish to remove the background from. Notice that the dialog has the title that we specified in the code.
12. Navigate to the rembg folder and give the file a name and set the file format to PNG. Click Save. In our example we save the file as les-no-bg.png.
13. You may encounter an error, but this is to be expected. Download the u2net file and save it to .u2net folder in your user directory. This folder is automatically created and stored.
14. Go back to Step 10 and re-run the code. There will be no error this time so skip step 13.
15. Go to the rembg folder and your image is now ready for use.
Complete Code Listing
from rembg import remove
from PIL import Image
import easygui as eg
input_path = eg.fileopenbox(title='Select image file')
output_path = eg.filesavebox(title='Save file to..')
input = Image.open(input_path)
output = remove(input)
output.save(output_path)
Python How Tos
- How To Install Python on Windows 10 and 11
- How to use For Loops in Python
- How to Enumerate in Python
- How to Create Executable Applications in Python
- How To Remove Backgrounds From Images With Python
- How to Create Web Apps with Python, HTML and Thonny
- How To Use Raspberry Pi Camera Module 3 with Python Code
Les Pounder is an associate editor at Tom's Hardware. He is a creative technologist and for seven years has created projects to educate and inspire minds both young and old. He has worked with the Raspberry Pi Foundation to write and deliver their teacher training program "Picademy".
-
LuaOle
What are the parameters/specifications for the background that is to be removed?Admin said:With just eight lines of Python code we can create an application to remove the background from many images.
How To Remove Backgrounds From Images With Python : Read more
Does it have to be mostly solid, mostly the same color, etc? 🤔 -
JohnD0e
just try it yourself and see. I tried it with a dog in front of a city park and it worked.LuaOle said:What are the parameters/specifications for the background that is to be removed?
Does it have to be mostly solid, mostly the same color, etc? 🤔