How to Create Executable Applications in Python
Build your own executable applications in Python
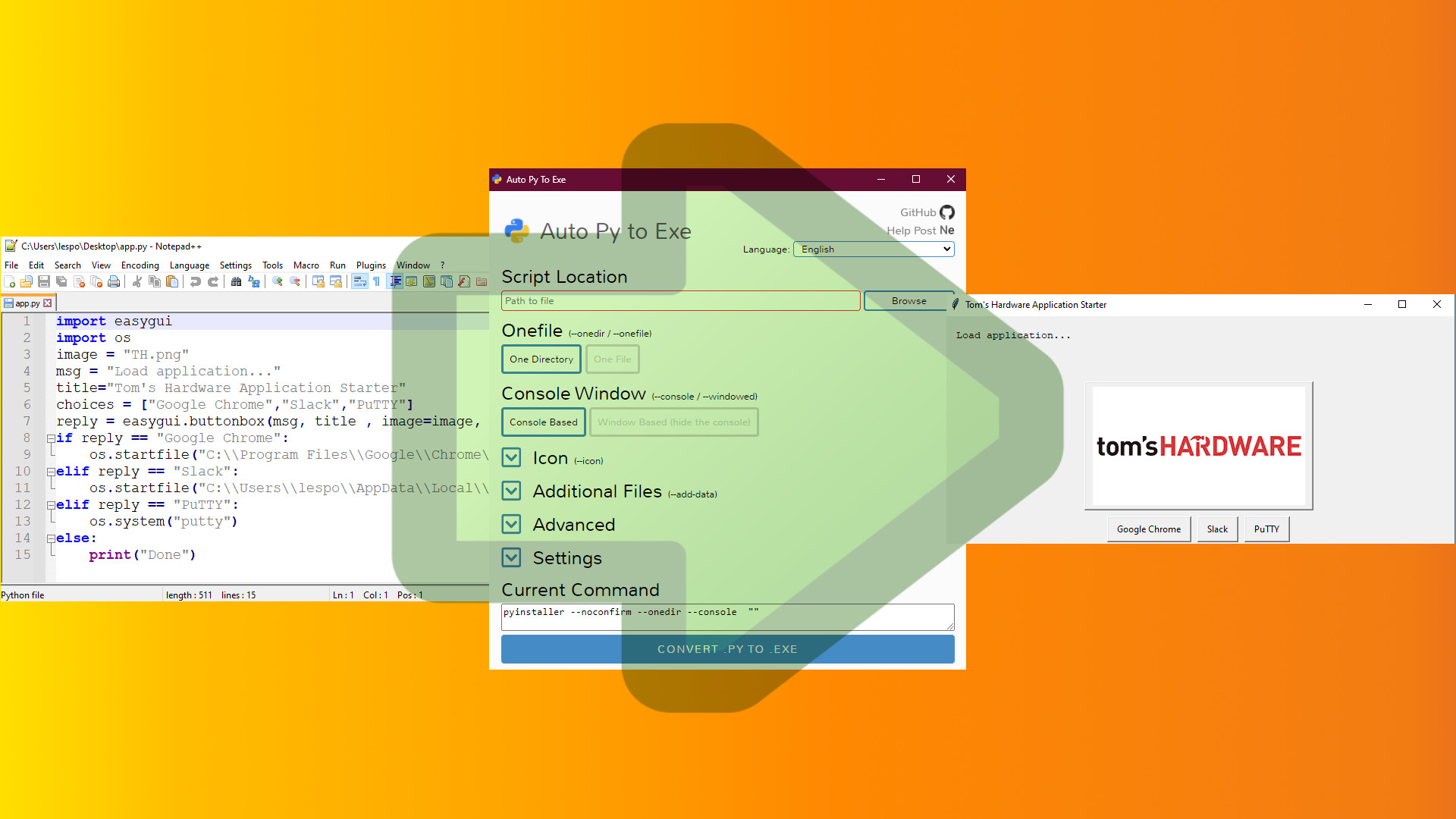
Used by NASA, ILM, Disney and hardware hackers, Python is a versatile programming language and an ideal choice for beginners. Whether you’re just creating a “Hello World” or a full-blown application, Python needs an interpreter and a bunch of supporting libraries to work. What if we could make a GUI application, all bundled inside of a single executable file?
With auto-py-to-exe ,a project by Brent Vollebregt we can easily create our own executable Python applications. Underneath the GUI is PyInstaller, a terminal based application to create Python executables for Windows, Mac and Linux. Veteran Pythonistas will be familiar with how PyInstaller works, but with auto-py-to-exe any user can easily create a single Python executable for their system.
In this how to, we are going to create a GUI Python application using EasyGUI, and then use auto-py-to-exe to create a standalone application that will run on any Microsoft Windows system, including systems without Python installed. Linux and Mac users will need to use the underlying PyInstaller command line tool. A simple app can be created using a single line instruction. By adding more arguments we can include icons, packaged libraries etc.
For example here is the code to create a onefile application using app.py as the project code.
pyinstaller --onefile app.py
Where auto-py-to-exe differs is that we have an easier means to create an application using a GUI tool.
How to Install auto-py-to-exe
1. Open a Command Prompt by searching for CMD.
2. Use the Python package manager pip to install auto-py-to-exe.
Get Tom's Hardware's best news and in-depth reviews, straight to your inbox.
pip install auto-py-to-exe
Create a Test Script
Our example application is a simple GUI to launch one of three applications. We use the EasyGUI Python library as it abstracts the complexities of creating a GUI application. All we need to provide is the logic that drives the application.
1. Open a PowerShell by right clicking on the Windows icon and selecting PowerShell.
2. Install EasyGUI using pip.
pip install easygui
3. Open a text editor to write the Python test script. We chose to use Notepad++, but you are free to use your favorite editor.
4. Import two Python modules, easygui and os. Easygui creates the GUI application and OS enables the code to interact with the operating system.
import easygui
import os
5. Create two variables, one for a message (msg) to the user while the other becomes the application title.
msg = "Load application..."
title="Tom's Hardware Application Starter"
6. Create a list, choices, and inside store three values which are the application names. Lists are Python’s arrays. Objects that can store multiple items. Each item has a numerical index, starting from zero.
choices = ["Google Chrome","Slack","PuTTY"]
7. Create an object, reply, to ask the user a question. In this case we use a button box from EasyGUI, each button is an option from the choices list. The chosen application is stored in the reply object.
reply = easygui.buttonbox(msg, title, choices=choices)
8. Use a conditional statement to read the value stored in reply and compare it to three conditions. The first checks reply to see if it contains “Google Chrome” if so it will open the Google Chrome browser. The startfile function requires the use of a full file path to the application. We need to use double \\ in the path as Python uses \ to insert illegal characters into a string.
if reply == "Google Chrome":
os.startfile("C:\\Program Files\\Google\\Chrome\\Application\\chrome.exe")
9. Use another conditional statement to check reply for slack.
elif reply == "Slack":
os.startfile("C:\\Users\\lespo\\AppData\\Local\\slack\\slack.exe")
10. Add another conditional statement to load PuTTY. Note that for PuTTY we use the os.system function as PuTTY is a registered app with the Windows path.
elif reply == "PuTTY":
os.system("putty")
11. Close the conditional test with an else condition to catch any other input.
else:
print("Done")
12. Save the file as app.py to the Desktop. If you are using an image in the application ensure that the image is also on the Desktop.
Complete Example Code Listing
import easygui
import os
msg = "Load application..."
title="Tom's Hardware Application Starter"
choices = ["Google Chrome","Slack","PuTTY"]
reply = easygui.buttonbox(msg, title , choices=choices)
if reply == "Google Chrome":
os.startfile("C:\\Program Files\\Google\\Chrome\\Application\\chrome.exe")
elif reply == "Slack":
os.startfile("C:\\Users\\lespo\\AppData\\Local\\slack\\slack.exe")
elif reply == "PuTTY":
os.system("putty")
else:
print("Done")
Using auto-py-to-exe
1. Open a Command Prompt by searching for CMD.
2. Run auto-py-to-exe from the prompt.
auto-py-to-exe
3. Click on Browse and navigate to our example Python file.
4. Set the application to use one file. This will condense the application and the supporting Python libraries into a single executable file.
5. Set the application to be Console Based. By doing this we will see any errors outputted to the Command Prompt. Once we are confident that the app works correctly, we can set this to Window Based.
6. Click on the Icon drop down and select an icon for your application. This is an optional step but it adds an extra level of quality to your application. Icons must be .ico files and we used a 64x64 pixel image as an icon.
7. Click on Advanced and, under –name, enter the name of your application. We chose App Launcher.
8. Scroll down and click on CONVERT .PY to .EXE to start the process. This will take a couple of minutes.
9. Click on Open Output Folder to open the folder containing the application.
10. Double click on the icon to run your application.
Python How Tos
- How To Install Python on Windows 10 and 11
- How to use For Loops in Python
- How to Enumerate in Python
- How to Create Executable Applications in Python
- How To Remove Backgrounds From Images With Python
- How to Create Web Apps with Python, HTML and Thonny
- How To Use Raspberry Pi Camera Module 3 with Python Code
Les Pounder is an associate editor at Tom's Hardware. He is a creative technologist and for seven years has created projects to educate and inspire minds both young and old. He has worked with the Raspberry Pi Foundation to write and deliver their teacher training program "Picademy".
-
Grobe The article only describes exe files for windows, so maybe change title to "How to Create Executable Applications for Windows in Python" ?Reply